How to create a successful CI/CD pipeline
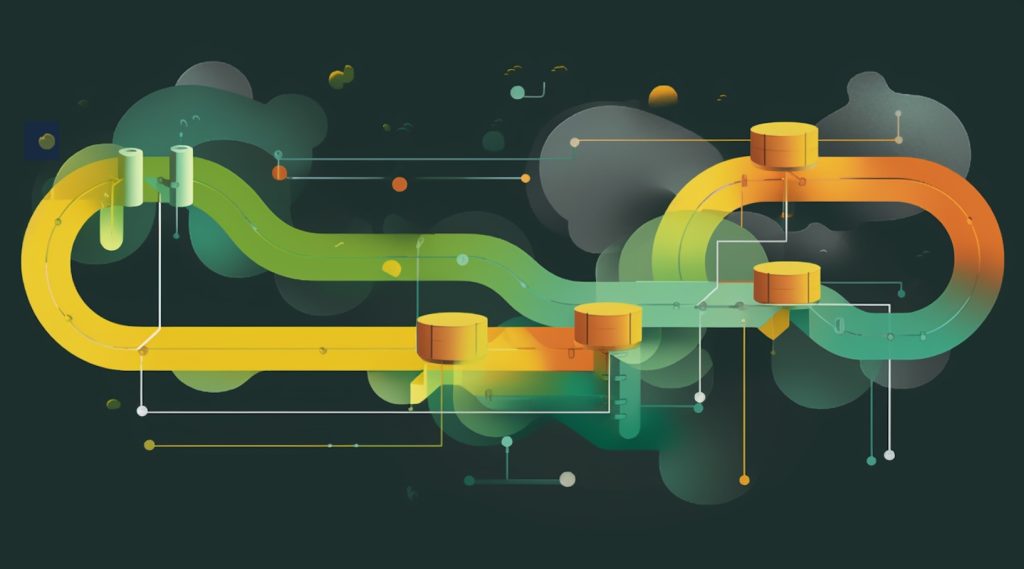
Outline:
- Introduction
- The Benefits of CI/CD
- Key Elements of a CI/CD Pipeline
- A practical example of how to Implement a Successful CI/CD Pipeline
- Common Challenges in Implementing CI/CD
- Tools and Technologies for CI/CD
- Conclusion

Introduction
In the world of software development, agility and speed are more important than ever before. To keep up with rapidly evolving user demands and market trends, development teams need to be able to deliver high-quality software quickly and efficiently. That’s where CI/CD comes in.
CI/CD, or continuous integration and continuous delivery, is a set of practices and tools that automate the build, testing, and deployment of software. By streamlining and automating these processes, teams can deliver software faster, with greater reliability and quality, while also improving collaboration and communication among team members.
This article will provide you with a comprehensive overview of why CI/CD is critical to modern software development and provide practical tips for implementing successful CI/CD pipelines. By highlighting the benefits of automation and providing real-world examples, this article will help you understand the value of CI/CD and how to make it work for your own development teams.
Benefits of CI/CD
- One of the main benefits of CI/CD is faster and more frequent delivery of software. With automated builds and testing, teams can quickly identify and fix issues, reducing the time it takes to release new features or bug fixes. This not only allows teams to respond more quickly to changing user demands or market trends, but it also helps them stay ahead of the competition.
- Another benefit of CI/CD is the improved quality and reliability of software. Automated testing catches issues earlier in the development process, reducing the risk of defects or bugs making it into production. This not only improves the user experience but also reduces the need for costly bug fixes and downtime.
- CI/CD also fosters better collaboration and communication among team members, as it requires regular and frequent interaction between developers, testers, and operations staff. This helps to break down silos and improve teamwork, while also fostering a culture of continuous improvement.
- In addition, CI/CD enables faster detection and resolution of issues, as automated testing and monitoring tools can quickly identify and notify teams of issues or performance problems. This allows teams to address issues proactively, reducing the risk of downtime or lost revenue.
- Finally, CI/CD provides greater visibility and transparency into the development process, allowing teams to track progress, measure performance, and identify areas for improvement.
Key elements of a CI/CD Pipeline
To implement a successful CI/CD pipeline, teams need to focus on several key elements, including:
- Source code management: a version control system such as Git or Subversion is critical.
- Automated builds: using tools such as Gradle, Maven, or Ant to automatically build and package the software.
- Automated testing: using testing frameworks such as JUnit, NUnit, or Selenium to automate testing, catch defects, and ensure quality.
- Continuous integration and delivery: using tools such as Jenkins, CircleCI, or Travis CI to automate the integration, testing, and delivery of software.
- Infrastructure as code: using tools such as Terraform, Ansible, or Puppet to automate the provisioning and management of infrastructure resources.
By combining these elements into a streamlined pipeline, teams can create a repeatable, scalable, and reliable process for delivering software.
Challenges and best practices for implementing CI/CD
While the benefits of CI/CD are clear, implementing a successful pipeline can be challenging. Common roadblocks include resistance to change, lack of skills or expertise, and organizational silos.
To overcome these challenges, teams can follow best practices such as:
- Start small and iterate: focus on one or two key areas to start and gradually expand the pipeline as needed.
- Embrace automation: automate as many tasks as possible, including testing, builds, and deployment.
- Foster a culture of collaboration and communication: break down silos between development, testing, and operations teams and encourage open communication and collaboration.
- Measure and monitor: track metrics such as build time, test coverage, and deployment frequency to measure performance and identify areas for improvement.
Example Implementation of CI/CD Pipeline
Let’s take an example of a web API developed using Node.js. The example implementation will use GitHub CI/CD for automation, as it is one of the most popular tools for implementing CI/CD pipelines through one of its features called GitHub Actions.
To follow along, you will need to clone this API build with NestJS. Code Repo
Setting up the repository
The first step in setting up a CI/CD pipeline using GitHub is to create a new repository for your project. Once the repository is created, you can add your code and any necessary files. You’ll also need to add a **README.md**
file that describes your project and a **.gitignore**
file that specifies any files or directories that should be excluded from version control.
In this article, we will be using the already created project you clone above from GitHub.
Creating a workflow
Next, you’ll need to create a workflow file that specifies the steps to be executed in your CI/CD pipeline. A workflow is a set of one or more jobs that are run sequentially or in parallel. Each job consists of one or more steps that perform specific actions, such as building and testing your code.
To create a workflow, you’ll need to create a **.github/workflows**
directory in your repository and add a YAML file with the workflow definition. For example, let’s create a simple workflow that builds and tests our code:
name: CI/CD Implementation - Deploy to S3
on:
push:
branches: [ main ]
jobs:
run:
runs-on: ubuntu-latest
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
steps:
- uses: actions/checkout@v2
- name: Install dependencies
run: yarn
- name: Lint
run: yarn lint
- name: Unit Test
run: yarn test
- name: Build
run: yarn build:dev
- name: Deploy
uses: reggionick/s3-deploy@v3
with:
folder: dist
bucket: ci-cd-tutorial-test
bucket-region: eu-east-1
dist-id: ETEST9NNL4431HD
invalidation: /*
delete-removed: true
no-cache: true
private: true
This code block is a YAML file that defines our workflow for a GitHub Action pipeline. It automates the process of deploying code to an S3 bucket:
Naming the workflow
This line specifies the name of the workflow. It is usually the first instruction for the YAML file.
name: CI/CD Implementation - Deploy to S3
Triggering the workflow
Once you’ve created your workflow, you’ll need to configure it to be triggered when certain events occur, such as a push to the repository or the creation of a pull request. In our example workflow, we’ve configured it to run when a push event occurs on the **main**
branch.
on:
push:
branches: [ main ]
GitHub Actions provides several events that you can use to trigger your workflows, such as **push**
, **pull_request**
, **schedule**
, and **workflow_dispatch**
. You can also use custom events to trigger your workflows based on external factors, such as a new release of a library or a change in an external API.
Setting workflow jobs and secrets
jobs:
run:
runs-on: ubuntu-latest
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
This section specifies the job that the workflow runs, the environment in which the job runs, and the AWS credentials that are needed to access the S3 bucket. This can be configured in GitHub secrets for security purposes and is needed to deploy our code to AWS s3. (You could skip the env
if you do not have an AWS account, this is only needed for Deploy
pipeline step.
Setting Workflow Steps
First, we checkout the code repository
steps:
- uses: actions/checkout@v2
Next, we install the dependencies required to run the application.
- name: Install dependencies
run: yarn
This step runs the linter to check the code for errors or issues.
- name: Unit Test
run: yarn test
This step runs the unit tests for the application.
- name: Build
run: yarn build:dev
This step builds the application for development.
- name: Deploy
uses: reggionick/s3-deploy@v3
with:
folder: dist
bucket: ci-cd-tutorial-test
bucket-region: eu-east-1
dist-id: ETEST9NNL4431HD
invalidation: /*
delete-removed: true
no-cache: true
private: true
This step deploys the code to the S3 bucket using the **s3-deploy**
GitHub Action. The **with**
keyword is used to specify the parameters for the deployment, including the folder to deploy (**dist**
), the name of the S3 bucket (**ci-cd-tutorial-test**
), the bucket region (**eu-east-1**
), and other optional parameters like cache settings and access permissions. (You could skip this step if you do not set up your AWS secrets)
Viewing the workflow results
After the workflow is triggered, you can view the results in the GitHub Actions tab of your repository. The Actions tab displays a list of all the workflows that have been triggered for your repository, along with the status of each workflow and the logs for each step.
If the workflow succeeds, you’ll see a green checkmark next to the workflow name. If the workflow fails, you’ll see a red X. You can click on the workflow name to view the details of the workflow, including the logs for each step.
Other tools and technologies for CI/CD
There are many tools and technologies available for implementing a CI/CD pipeline. Some of the most popular include:
- Jenkins: an open-source automation server that provides hundreds of plugins for building, testing, and deploying software.
- Travis CI: a cloud-based CI/CD platform that automates builds and testing for GitHub projects.
- CircleCI: a cloud-based CI/CD platform that provides fast, reliable builds and testing for projects hosted on GitHub, Bitbucket, or GitLab.
- GitLab CI/CD: an integrated CI/CD platform that provides automated testing, builds, and deployments.
- AWS CodePipeline: a fully managed CI/CD service that automates the release of software changes.
- Google Cloud Build: a fully managed CI/CD service that provides fast, reliable builds for Google Cloud Platform projects.
Each tool has its own strengths and weaknesses, and teams should carefully evaluate their needs and requirements before choosing a tool.
Extending the CI/CD pipeline with multiple tools
To better understand how CI/CD pipeline will work with more tools, let’s take a look at an example. Let’s say we have a simple web application built with Node.js and hosted on GitHub. Here’s how we could set up a CI/CD pipeline for this project:
- Code repository: We’ll use GitHub as our code repository. All code changes will be pushed to this repository.
- Continuous Integration: We’ll use CircleCI for our continuous integration tool. We’ll configure CircleCI to build the application every time new code is pushed to the repository. CircleCI will run tests to ensure that the code is correct and meets our quality standards.
- Code Quality: We’ll use SonarQube to measure the quality of the code. SonarQube will analyze the code and provide a report on any issues or potential issues. This will help us catch bugs early in the development process.
- Continuous Delivery: We’ll use AWS CodeDeploy for continuous delivery. Once the code passes all tests and quality checks, CodeDeploy will automatically deploy the code to our staging environment.
- Testing: We’ll use Selenium for testing the application. Selenium will simulate user interactions with the web application and ensure that it works as expected.
- Continuous Deployment: Once the application passes all tests, CodeDeploy will automatically deploy it to the production environment.
By setting up this pipeline, we can ensure that any changes to the code are thoroughly tested and meet our quality standards before they’re deployed to production. We can also catch and fix any issues early in the development process, which saves time and reduces the risk of bugs making their way into production.
Conclusion
CI/CD is critical to modern software development, providing a way for teams to deliver software quickly, reliably, and with high quality. By automating key tasks such as testing, builds, and deployment, teams can reduce the risk of defects and bugs, while also improving collaboration and communication among team members.
To implement a successful CI/CD pipeline, teams need to focus on critical elements such as source code management, automated testing, and infrastructure as code, while also following best practices such as starting small, embracing automation, fostering collaboration, and measuring performance.
With the right tools and practices in place, teams can create a streamlined and scalable pipeline for delivering software that meets the needs of their users and stays ahead of the competition.
Aviator: Automate your cumbersome merge processes

Aviator automates tedious developer workflows by managing git Pull Requests (PRs) and continuous integration test (CI) runs to help your team avoid broken builds, streamline cumbersome merge processes, manage cross-PR dependencies, and handle flaky tests while maintaining their security compliance.
There are 4 key components to Aviator:
- MergeQueue – an automated queue that manages the merging workflow for your GitHub repository to help protect important branches from broken builds. The Aviator bot uses GitHub Labels to identify Pull Requests (PRs) that are ready to be merged, validates CI checks, processes semantic conflicts, and merges the PRs automatically.
- ChangeSets – workflows to synchronize validating and merging multiple PRs within the same repository or multiple repositories. Useful when your team often sees groups of related PRs that need to be merged together, or otherwise treated as a single broader unit of change.
- TestDeck – a tool to automatically detect, take action on, and process results from flaky tests in your CI infrastructure.
- Stacked PRs CLI – a command line tool that helps developers manage cross-PR dependencies. This tool also automates syncing and merging of stacked PRs. Useful when your team wants to promote a culture of smaller, incremental PRs instead of large changes, or when your workflows involve keeping multiple, dependent PRs in sync.