Java Version Upgrade: A Hands-on Guide from Java 8 to 17
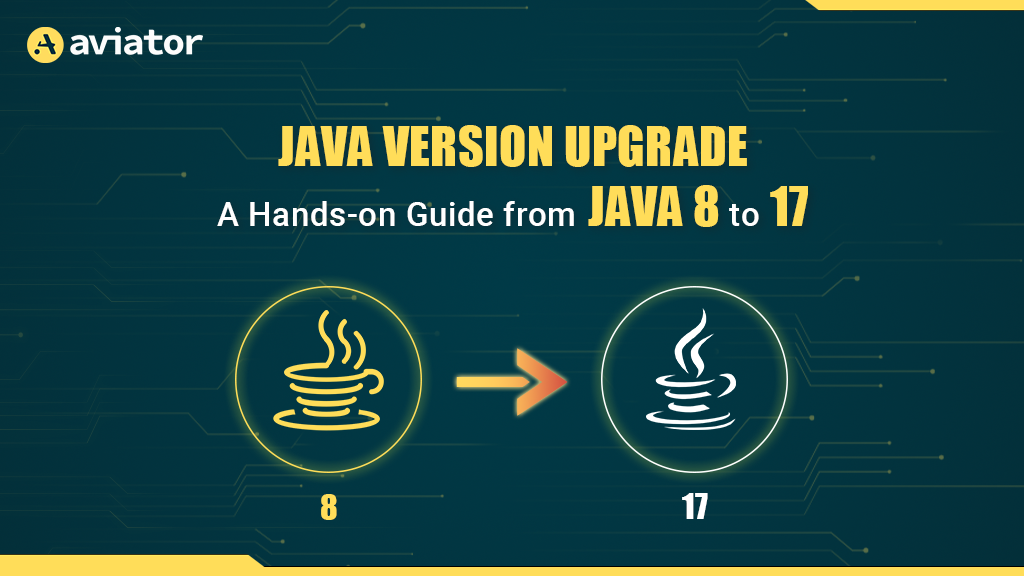
Upgrading Java might seem dangerous, especially if your version “just works.” But keeping Java 8 in 2025 means bypassing significant performance gains, up-to-date syntax, and extended support. Considering a Java 8 upgrade now will allow you to benefit from these improvements.
Imagine you’re working on a backend system built in Java 8—a stable monolith that’s served the business well for years. But now, CI builds drag, memory usage spikes under load, and new developers struggle to maintain legacy patterns. Meanwhile, frameworks like Spring Boot 3, JUnit 5, and observability tools such as OpenTelemetry and Micrometer no longer support Java 8. Staying put is no longer sustainable.
This tutorial takes you through the what, why, and how of upgrading from Java 8 to Java 17. We delve into important language features, performance improvements, breaking changes, and migration strategies, with real code examples. Whether it’s maintaining an old code base or updating the Java version for your backend stack, this blog helps you to plan and implement smoothly with confidence.
Upgrading Java: The What, Why, and Now
, It has been more than ten years now since Java 8 launched in 2014, and whereas it’s still reliable, but it’s no longer the recommended default.
For developers looking to upgrade, understanding the differences highlighted by a comparison of Java 8 vs Java 17 is crucial.
LTS Releases Give You a Safer Path Forward
Oracle and other vendors like Azul, Amazon, and Eclipse Foundation mark certain Java versions as Long-Term Support (LTS). These get security patches and updates for at least 4-8 years, making them ideal for production environments.
- Java 11 (released in 2018) is the first LTS after Java 8, following two non-LTS releases (Java 9 and Java 10).
- Java 17 (released in 2021) is the next LTS and current go-to for many enterprises.
Modern Java Is Easier to Write
Upgrading isn’t just about using the latest version—it’s about gaining access to powerful language features, better performance, stronger security, and modern development tools that simplify code and boost productivity.
- var: Introduced in Java 10, helps reduce boilerplate in local variable declarations. It doesn’t compromise type safety—it simply infers the type from the assigned value at compile time.
- records: Introduced in Java 14 (preview) and finalized in Java 16, they simplify DTOs and value classes with less code and more clarity.
- Sealed classes: Introduced in Java 17, sealed classes give you fine-grained control over inheritance by explicitly declaring which classes can extend or implement them. This helps enforce architectural boundaries and makes code easier to reason about.
These modern constructs make code more expressive, compact, and maintainable.
Now let’s check what the issues are with modern frameworks.
Compatibility with Modern Tooling and Frameworks
Frameworks like Spring Boot, Micronaut, and Quarkus have started targeting newer Java versions for optimal performance. Many modern CI/CD tools like Jenkins, Gitlab, testing libraries like JUnit 5, Mockito, and observability stacks like Micrometer, Prometheus drop support for Java 8 as they adopt newer JVM features.
Breaking Changes and Migration Considerations
Upgrading from Java 8 to 17 isn’t a drop-in replacement. Understanding what’s deprecated, removed, or restructured helps prevent build failures and runtime surprises.
Removed Modules That Might Break Your Build
Java 9 introduced the Java Platform Module System (JPMS), and with it came modularization and removals.
Some commonly used modules in Java 8 were either deprecated or entirely removed by Java 11:
- java.xml.bind (JAXB)
Used for XML serialization/deserialization. Removed in Java 11.
Fix: Add it as a separate dependency: javax.xml.bind:jaxb-api. - Nashorn JavaScript Engine
Deprecated in Java 11 and removed in Java 15.
Fix: Use alternatives like GraalVM JavaScript if scripting is required. - CORBA, Java EE modules (java.activation, java.xml.ws, etc.)
All have been removed.
Fix: These need external libraries now, often via Maven or Gradle.
Impact on Build Tools and Plugin Compatibility
Your build system might be coupled with Java 8-specific tooling or plugin versions. Migration requires checking compatibility across your stack:
- Maven
- Ensure you’re on Maven 3.8+ for full Java 17 compatibility.
Update compiler plugin:
<maven-compiler-plugin>
<configuration>
<release>17</release>
</configuration>
</maven-compiler-plugin>
- Gradle
- Use Gradle 7+ for Java 17 support.
- Update the toolchain or javaVersion block accordingly.
- Use Gradle 7+ for Java 17 support.
Many older plugin versions (checkstyle, spotbugs, surefire) may not support Java 17. Make sure you audit and upgrade them too.
What’s New in Java 17 (Compared to Java 8)
Upgrading from Java 8 to 17 is more than just ticking a version number, it’s a leap across almost a decade of innovation. Java has evolved to make developers more productive, code more expressive, and applications more performant.
Let’s break down what’s changed between Java 9 and 17, grouped by themes and versions.
Java 9–11: Foundational Shifts
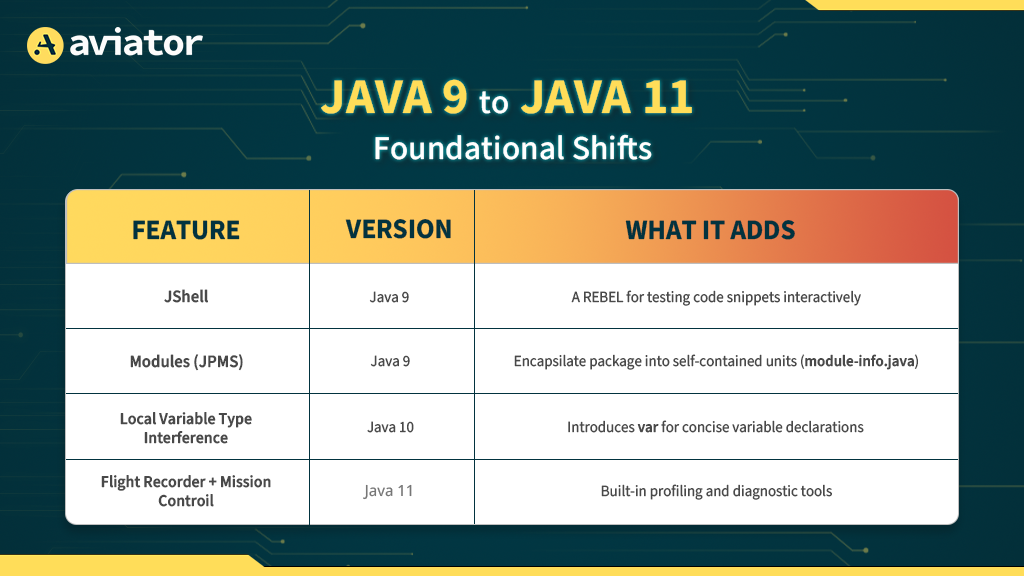
Now, as we are done with the feature shits and upgrade, let’s go through example for each.
Example: Using var to simplify verbose declarations
// Java 8
Map<String, List<Integer>> map = new HashMap<>();
// Java 10+
var map = new HashMap<String, List<Integer>>();
Java 12–14: Expressive Syntax Improvements
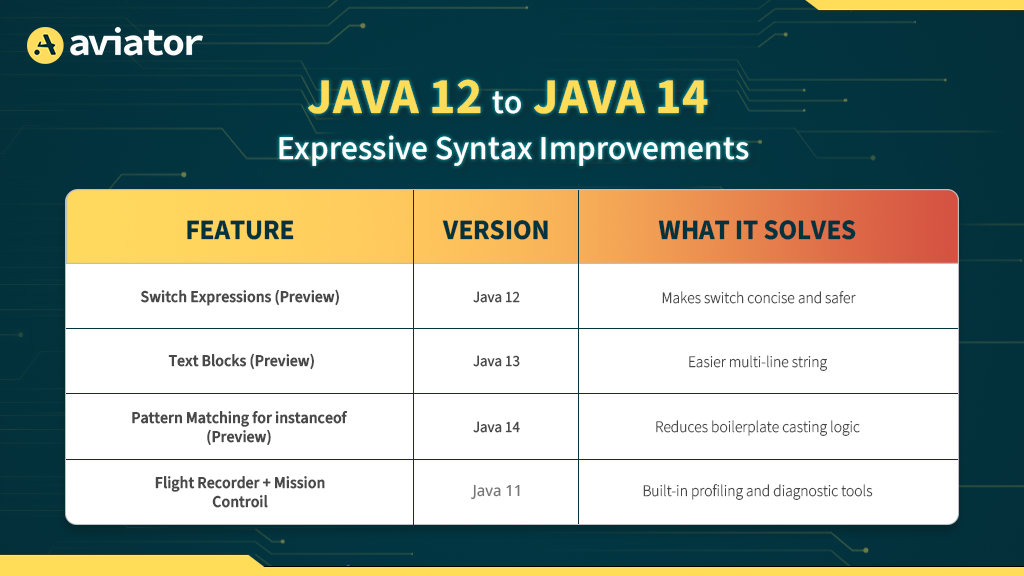
Example: Modern switch expression
// Java 8 traditional switch statement
int result;
switch (value) {
case "A":
result = 1; // Assign value for case "A"
break; // Prevent fall-through
default:
result = 0; // Default case assignment
}
// Java 12+ switch expression (concise and returns a value)
int result = switch (value) {
case "A" -> 1; // Directly returns 1 for case "A"
default -> 0; // Returns 0 for any other value
};
Java 15–17: Concise Models, Safer APIs
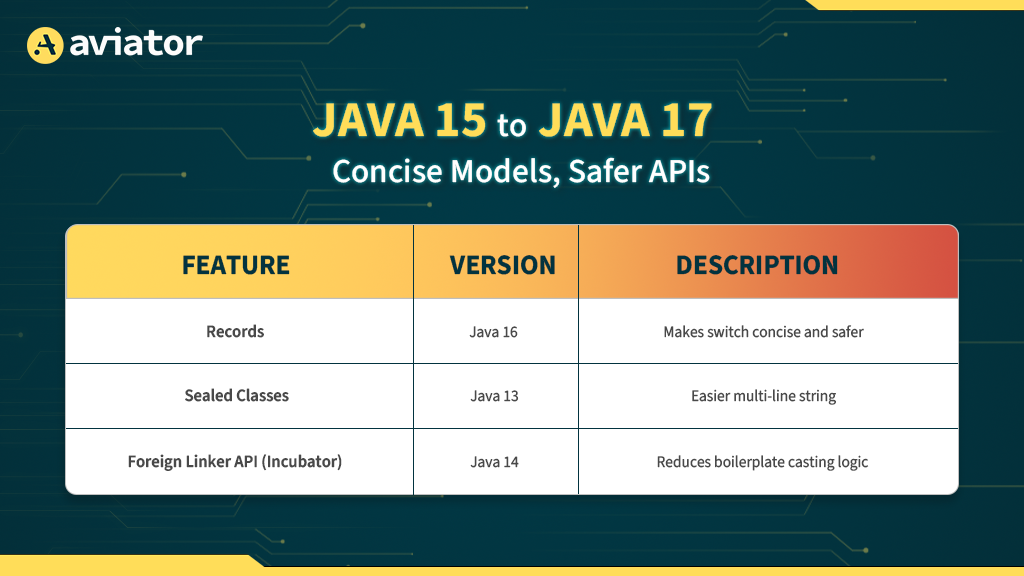
Example: Replacing boilerplate POJOs with Records
// Java 8 version
public class User {
// A private final field to store the user's name
private final String name;
// Constructor to initialize the name
public User(String name) {
this.name = name;
}
// Getter method to access the name
public String getName() {
return name;
}
}
// Java 16+ version using records
// 'record' automatically creates:
// - private final fields
// - constructor
// - getters (named exactly like the field, i.e., user.name())
// - equals(), hashCode(), and toString() methods
record User(String name) {}
Migration Workflow: Java 8 to 17
If you’re wondering, “How do I upgrade Java?”, this guide is for you. Here’s a step-by-step workflow you can follow to make the upgrade smooth, predictable, and safe.
Step 1: Prepare the Codebase
- Clean up old dependencies: Identify deprecated or Java EE modules removed in 11+.
- Run static analysis: Use tools like SpotBugs, PMD, or SonarQube to catch risky constructs.
- Add compiler flags: Configure your build tool to specify the source and target compatibility using –release or appropriate java.version.
<!-- Maven compiler plugin example -->
<configuration>
<release>8</release>
</configuration>
Step 2: Upgrade Your Build System
- Update Maven or Gradle to recent versions that support Java 17 (e.g., Maven 3.8+, Gradle 7+).
- Use toolchains to target multiple JDKs for hybrid compatibility testing.
- Set cross-compilation flags like –release 8 to ensure Java 8 compatibility during migration.
Step 3: Modernize Gradually
- Enable error-prone or checkstyle rules to enforce modern Java usage patterns.
- Refactor incrementally: Start with syntax-only upgrades like text blocks or var declarations.
Example:
Before (Java 8):
String json = "{\n" +
" \"name\": \"Ainan\",\n" +
" \"role\": \"Developer\"\n" +
"}";
After (Java 15+ with Text Blocks):
String json = """
{
"name": "Ainan",
"role": "Developer"
}
""";
- Test each feature in isolation, avoid sweeping refactors.
Step 4: Validate Compatibility
- Test builds across JDKs (8, 11, and 17) using CI.
- Run jdeps to find the modules your code depends on.
- Profile runtime behavior using Java Flight Recorder (JFR) to catch regressions.
Step 5: Deploying with Java 17
- Use Canary or Blue-Green deployments to test with a small subset of traffic first.
- Implement rollback strategies with Docker containers or JDK isolation.
- Monitor performance and error logs closely during rollout.
Now let’s go through the example migration setup.
Example Of Migration Setup as per the Workflow
Migrating a Spring Boot App from Java 8 to 17
To bring a Spring Boot project up to Java 17, a few strategic updates are needed, starting with your build configuration and environment setup.
pom.xml Changes (Maven Toolchain Support)
<properties>
<maven.compiler.release>17</maven.compiler.release>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-toolchains-plugin</artifactId>
<version>3.1.1</version>
<executions>
<execution>
<goals>
<goal>toolchain</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Setting Up JDK 17 in a Dockerfile
Use the official Eclipse Temurin base image to containerize your app with Java 17:
FROM eclipse-temurin:17-jdk
WORKDIR /app
COPY . .
RUN ./mvnw clean install
CMD ["java", "-jar", "target/app.jar"]
GitHub Actions for Java 17
Automate your builds and tests with Java 17 support:
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 17
uses: actions/setup-java@v3
with:
java-version: '17'
distribution: 'temurin'
- run: ./mvnw clean test
JVM Tuning for ZGC or G1
Update your startup script:
ZGC offers ultra-low pause times, ideal for latency-sensitive services:
java -XX:+UseZGC -Xms512m -Xmx2g -jar app.jar
Or for G1 (default in JDK 9+):
G1 is a balanced collector with predictable pauses:
java -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -jar app.jar
Testing Modern Features
Records to Reduce Boilerplate
Records auto-generate constructors, getters, and more:
// Old Java 8 style
public class Person {
private final String name;
public Person(String name) { this.name = name; }
public String getName() { return name; }
}
// Java 17 record
record Person(String name) {}
Pattern Matching with instanceof
No more redundant casting:
// Java 8
if (obj instanceof String) {
String s = (String) obj;
System.out.println(s.toUpperCase());
}
// Java 17
if (obj instanceof String s) {
System.out.println(s.toUpperCase());
}
Switch Expressions
More expressive, less error-prone:
// Java 8
String result;
switch (status) {
case "START": result = "begin"; break;
case "STOP": result = "end"; break;
default: result = "unknown";
}
// Java 17
String result = switch (status) {
case "START" -> "begin";
case "STOP" -> "end";
default -> "unknown";
};
Conclusion
Upgrading to Java 17 from Java 8 is a wise move, bringing long-term advantages. You receive better security, performance, and a much more expressive language. But most importantly, you future-proof your applications with LTS support and compatibility with new frameworks and tools.
Whether you’re migrating a microservice or a monolith, breaking the upgrade into manageable steps helps avoid risk while embracing all the goodness Java has picked up in the last decade.
FAQs
1. Why upgrade from Java 8 to 17?
Upgrading from Java 8 to Java 17 delivers performance enhancements, new language capabilities, and enhanced tooling support. It’s a strategic upgrade that promises stability and long-term growth.
2. What is the difference between Java 8 and Java 17?
Considering the difference between Java 8 and Java 17, Java 17 has features like var, records, text blocks, sealed classes, enhanced garbage collection (ZGC, G1), new APIs, and removal of legacy modules. It is leaner, faster, and simpler to maintain than Java 8.
3. Which Java version is faster?
Each successive release of Java improves performance, but Java 17 is faster than Java 8 because there are smarter JIT optimizations, better memory management, and better garbage collection. Java 21 and 22 continue the trend, and Java 24 (which comes out now) takes it up a notch.
4. Which Java version is better, 17 or 21?
Both are great, but Java 17 is an LTS release and thus the safer default for enterprise environments. Java 21 is also an LTS and includes more recent features like virtual threads. Choose based on your support needs and plan.